Flizzy Burd ๐ฆ is an exciting 2D platformer ๐ฎ where players take control of a brave little bird navigating through an endless world ๐ filled with challenges. The bird must flap its wings ๐ชฝ at the right time โฑ๏ธ to avoid obstacles ๐๏ธ and stay alive ๐ฅ.
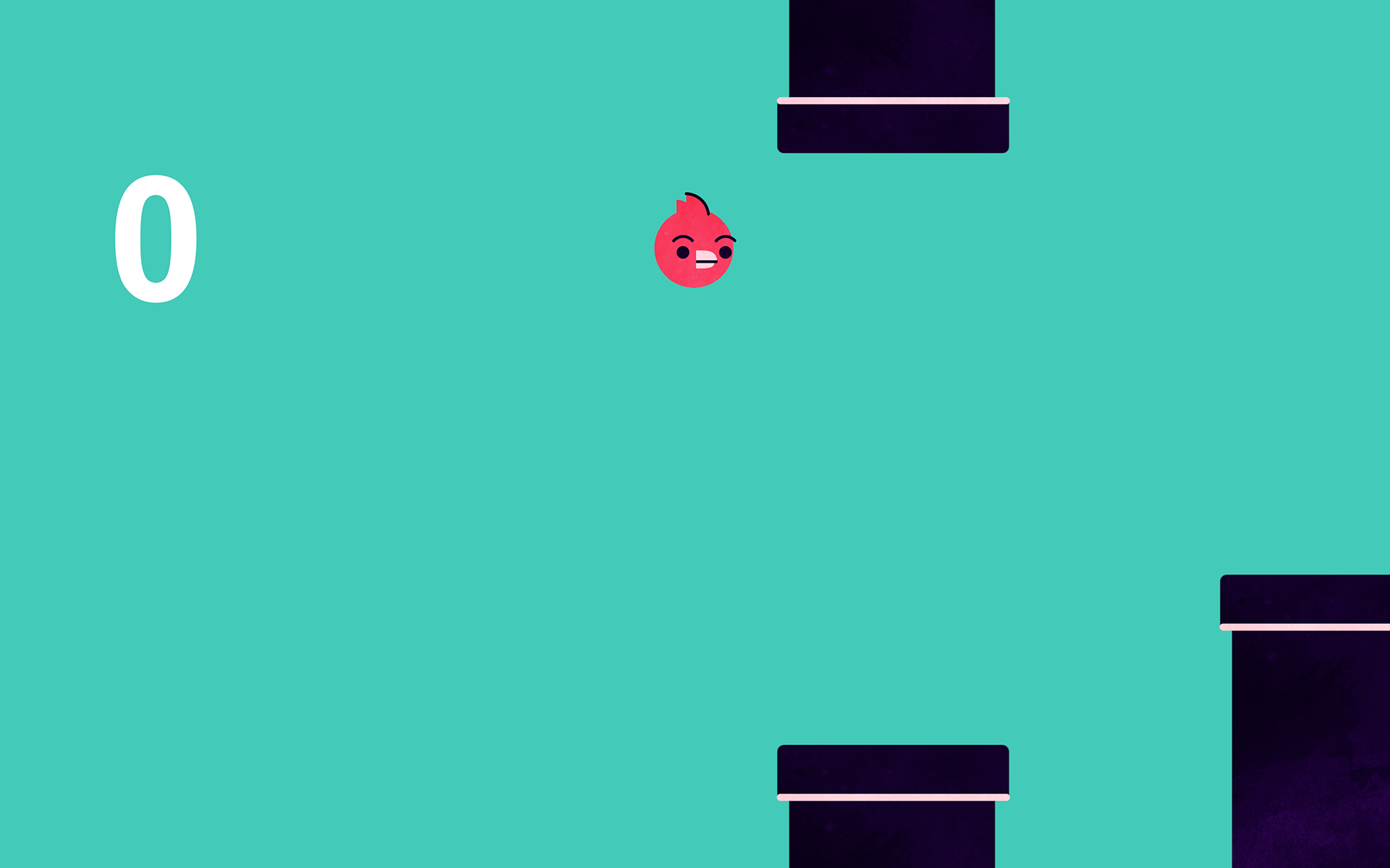
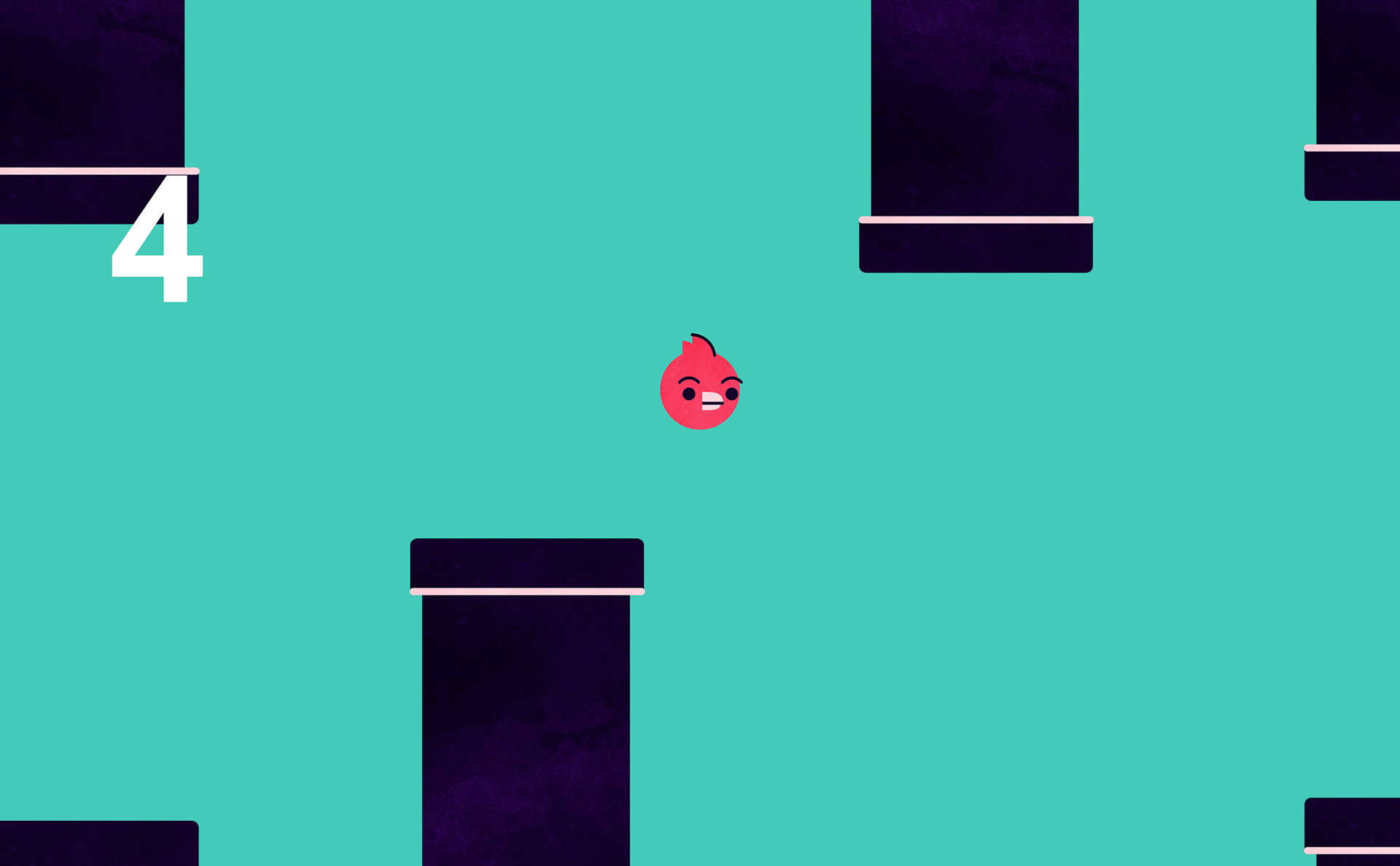
๐ฆBird Script๐ฆ
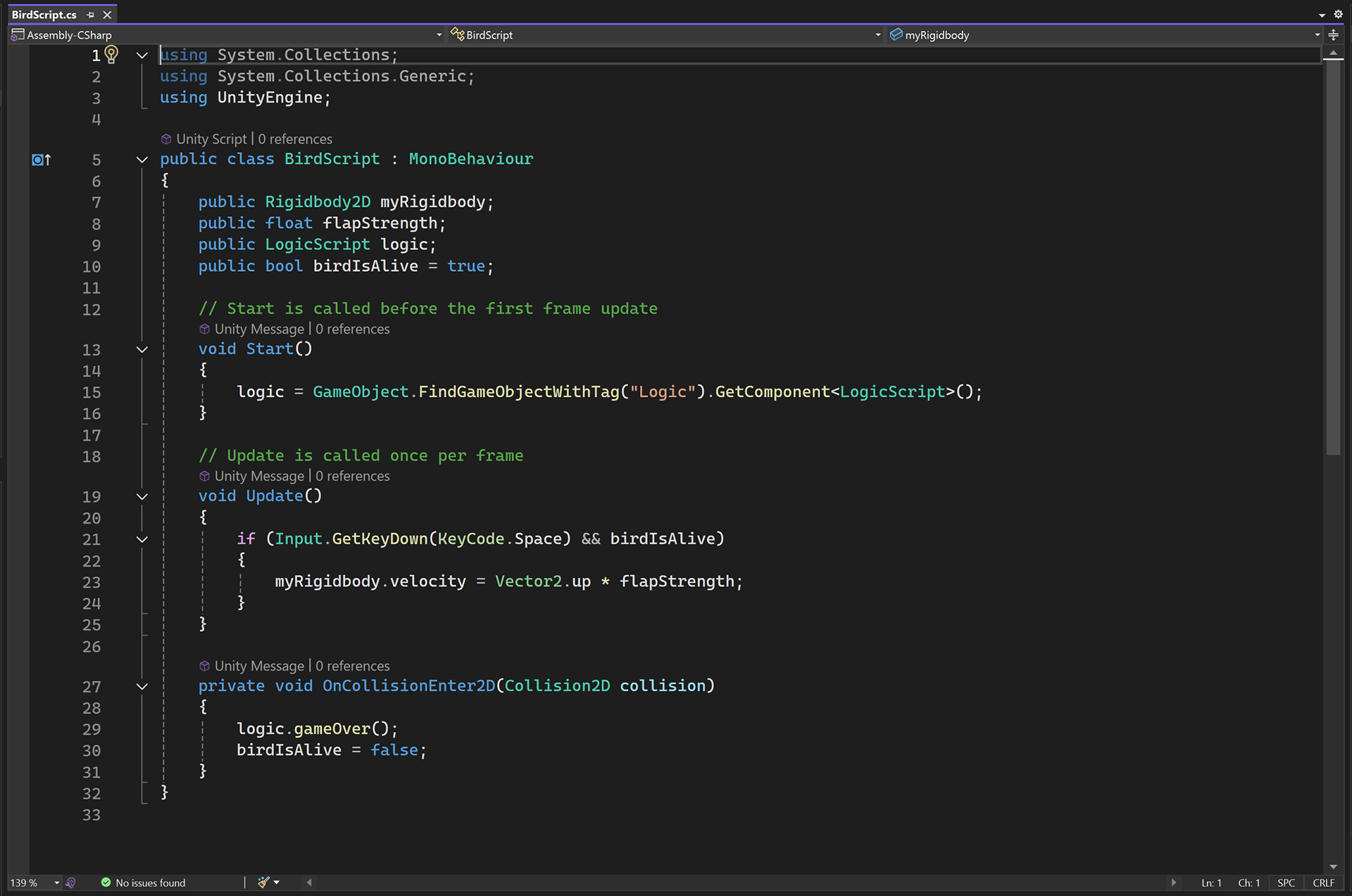
1. VARIABLES:
- myRigidbody: A reference to the birdโs Rigidbody2D component (handles the bird's physics ๐งฒ, gravity ๐, and movement ๐โโ๏ธ).
- flapStrength: The strength ๐ช of the bird's flap ๐ฆ
(how high the bird flies when the spacebar is pressed โฌ๏ธ).
- logic: A reference to the LogicScript ๐, which controls the gameโs logic (like checking if the game is over ๐จ).
- birdIsAlive: A boolean ๐ that tracks whether the bird is still alive ๐ฆโค๏ธ or has crashed ๐ชฆ.
2. START METHOD:
- Sets logic to the LogicScript (finds the object in the scene using the "Logic" tag ๐ท๏ธ) so the bird can interact with the gameโs logic (like game-over checks and scoring ๐ฏ).
3. UPDATE METHOD:
- Called every frame โฑ๏ธ to check if the player presses the spacebar (flap button ๐ฆ
). If the bird is still alive (birdIsAlive is true ๐ฆโ
), it applies an upward force โฌ๏ธ to the Rigidbody2D (making the bird "flap" ๐ฆ๐จ).
- The flapStrength determines how high the bird will fly with each flap ๐.
4. ONCOLLISIONENTER2D METHOD:
- Triggered when the bird collides ๐ with another object (e.g., a pipe ๐๏ธ or obstacle ๐ชต).
- When a collision happens, it calls the gameOver() method from the LogicScript ๐น๏ธ to end the game ๐ and sets birdIsAlive to false โ, stopping the bird's movement ๐ฆ๐.
IN SUMMARY:
The BirdScript makes the bird flap ๐ฆ
when the player presses the spacebar โฌ๏ธ. If the bird crashes ๐ into anything (like an obstacle ๐๏ธ), it triggers a game-over sequence ๐จ, stopping the game ๐. It's a basic script for handling player input (flapping ๐ฆ) and detecting collisions (game over ๐).
Logic Script
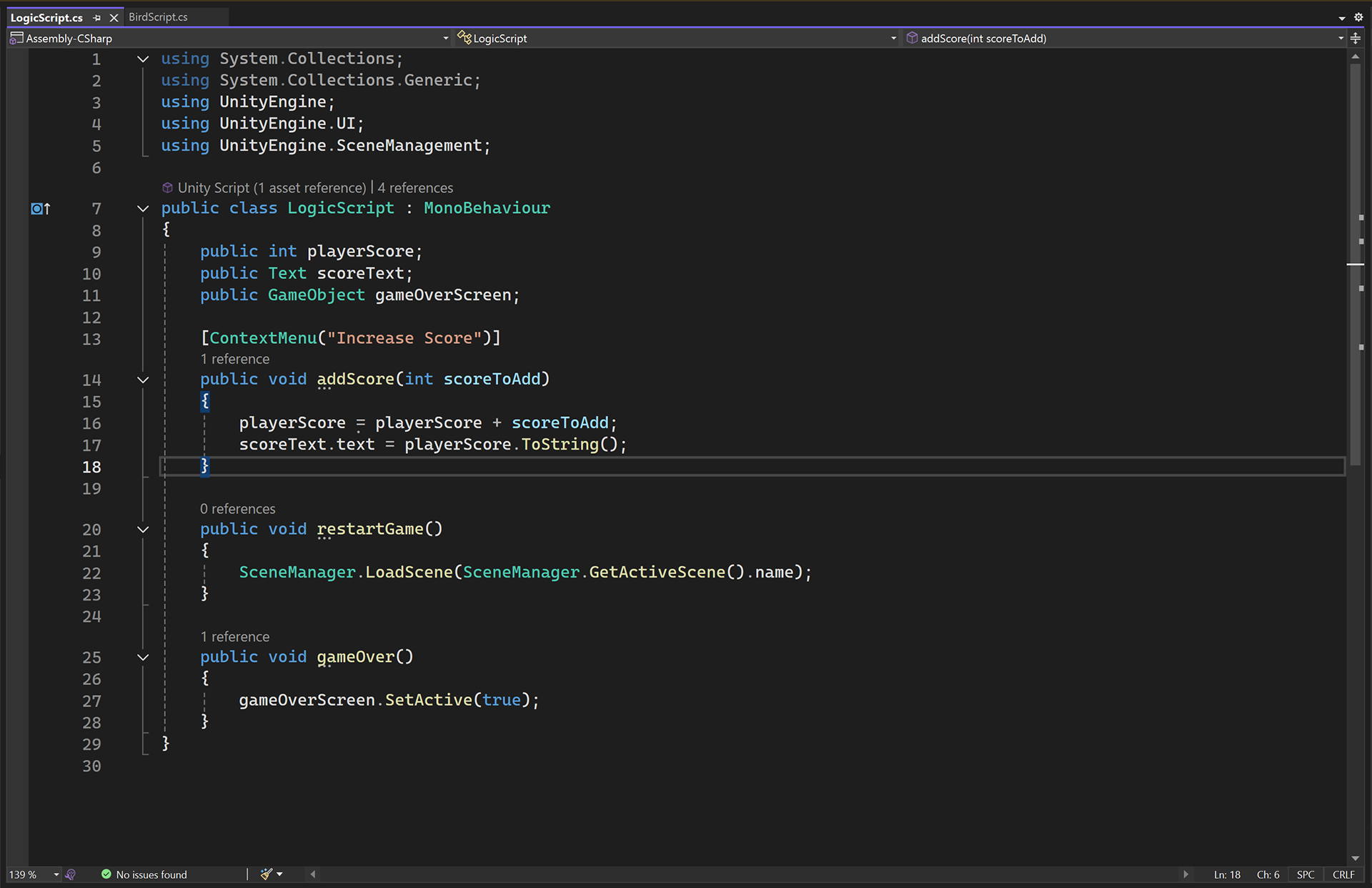
WHAT IT DOES:
- This script manages the game logic for scoring ๐ฏ, restarting ๐, and handling the game over ๐ state in Flizzy Burd.
FEATURES:
- addScore(int scoreToAdd): Increases the player's score ๐ฏ by the given amount โ and updates the score displayed on the screen ๐ฅ๏ธ.
- restartGame(): Reloads the current scene ๐ to restart the game, giving the player a fresh start ๐ฑ.
- gameOver(): Displays the game over screen ๐ when the game is over ๐, signaling the end of the bird's journey ๐ถโโ๏ธ๐จ.
- The script keeps track of the playerโs score ๐ฏ, displays it in the UI ๐ฅ๏ธ, and provides functions to restart the game ๐ or show a game over screen ๐ when the birdโs flight ends.
Pipe Middleย Script
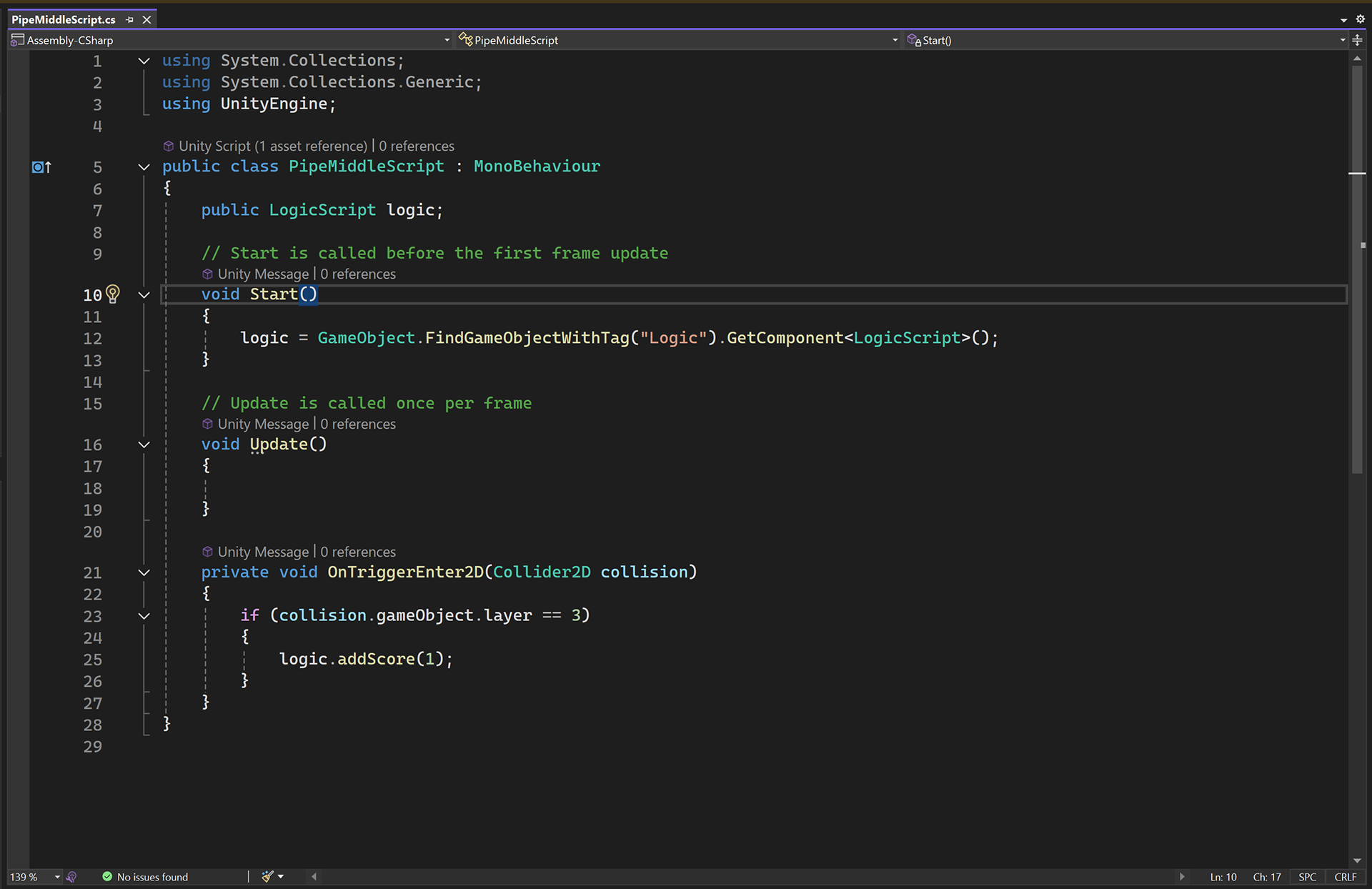
WHAT IT DOES:
- This script manages the scoring logic ๐ when the bird ๐ฆ passes through the middle of a pipe ๐ง in Flizzy Burd. Here's what each part does:
- Start(): It finds the LogicScript attached to the game object tagged with "Logic" ๐ and stores it in the logic variable ๐ฅ.
- OnTriggerEnter2D(Collider2D collision): This method checks when the bird ๐ฆ passes through the middle of the pipe ๐ง (the "trigger" area).
- If the bird (on layer 3) ๐ฆ collides with the pipe ๐ง, it calls the addScore(1) function from the LogicScript ๐ฏ, increasing the score by 1 โ.
- This script ensures that the player earns points ๐ฏ whenever the bird ๐ฆ successfully passes through a pipe ๐ง, adding to the challenge and excitement of Flizzy Burd!
Pipe Move Script
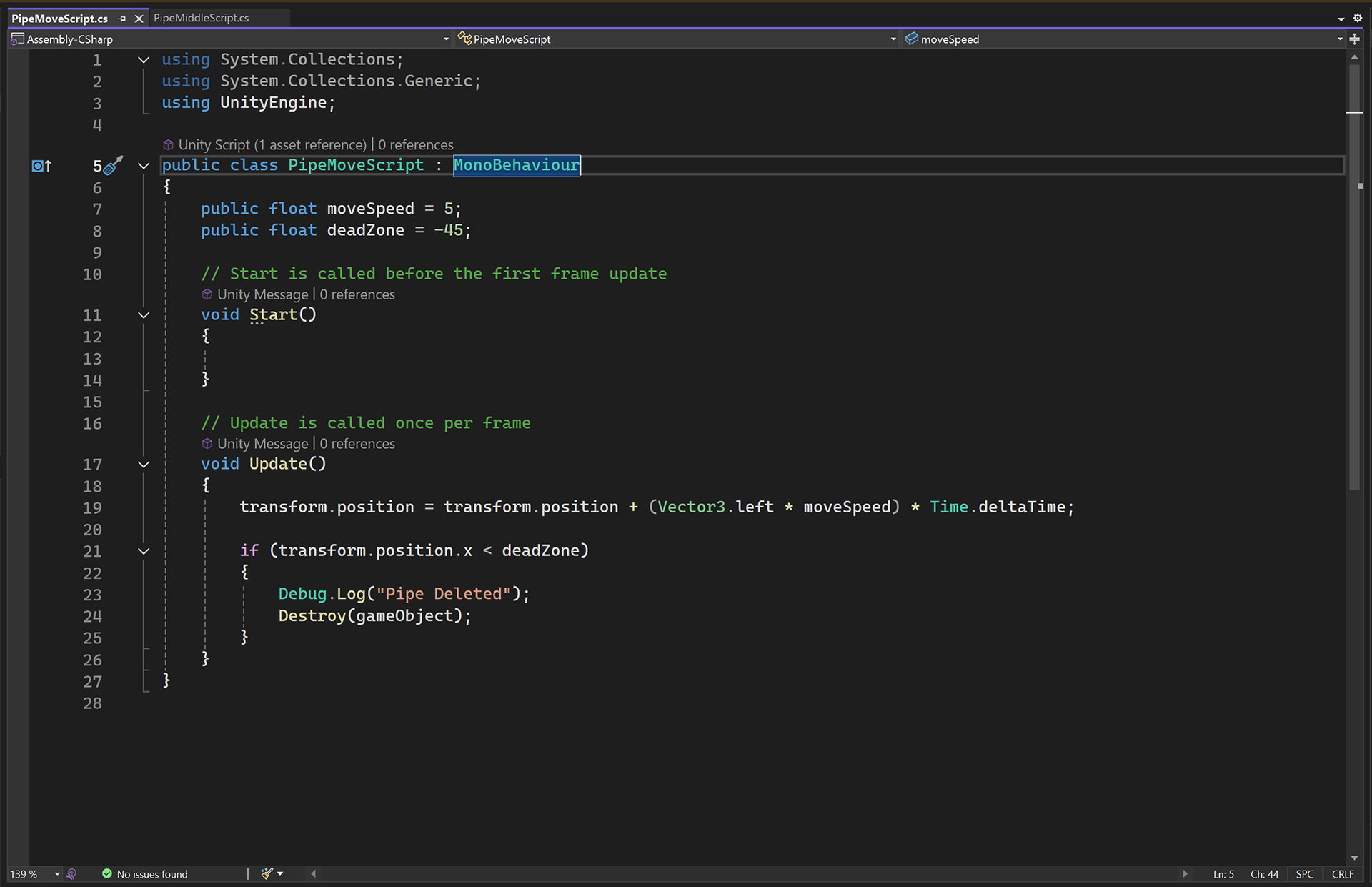
WHAT IT DOES:
This script handles the movement ๐โโ๏ธ and deletion ๐ฅ of pipes ๐ง in Flizzy Burd. Here's what each part does:
- moveSpeed: Controls how fast the pipe ๐ง moves across the screen โฉ.
- deadZone: The X position ๐บ๏ธ at which the pipe ๐ง is destroyed ๐ฅ.
KEY ACTIONS:
Update():
- Moves the pipe ๐ง to the left across the screen โฌ
๏ธ at a speed determined by moveSpeed.
- If the pipe ๐ง moves past the deadZone (a specific X position), it gets destroyed ๐ฅ using Destroy(gameObject).
This script ensures the pipes ๐ง move across the screen and are deleted when they exit the visible area ๐, preventing them from cluttering the game world ๐ช๏ธ.
Pipe Spawn Script
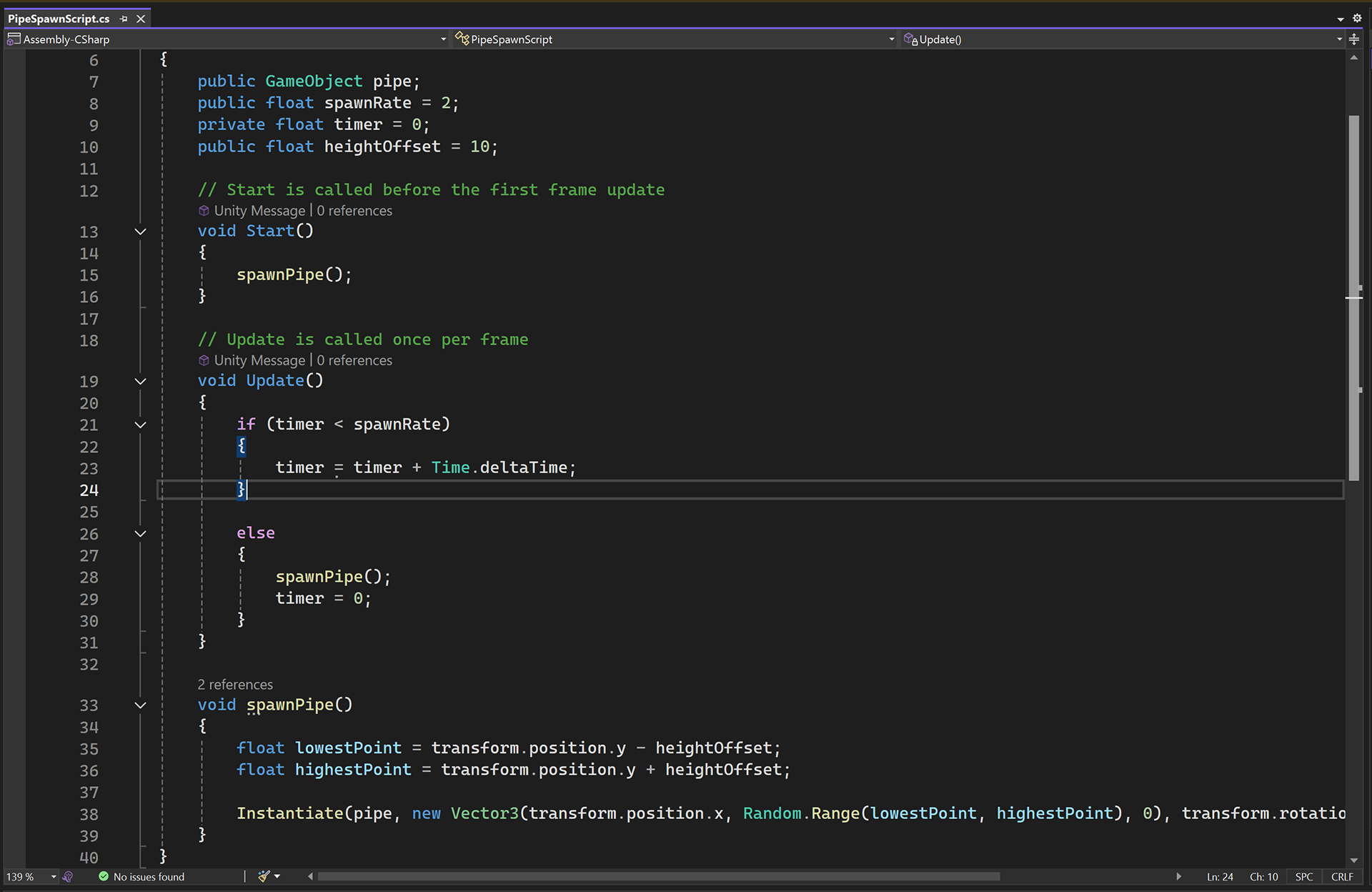
WHAT IT DOES:
This script spawns pipes ๐ง in Flizzy Burd at regular intervals. Here's what each part does:
- pipe: The pipe ๐ง prefab that gets instantiated (spawned) in the game world.
- spawnRate: The time ๐ between each pipe spawn. Default is 2 seconds.
- timer: Keeps track of the time passed and triggers a new spawn when it reaches spawnRate.
- heightOffset: Determines how far the pipes ๐ฎ can spawn from the origin point (higher or lower).
KEY ACTIONS:
Start():
- Calls the spawnPipe() function to spawn the first pipe ๐ง when the game starts ๐ฎ.
Update():
- Checks if the timer is less than the spawnRate. If true, it adds time ๐ to the timer.
- If the timer exceeds the spawnRate, it calls spawnPipe() to spawn a new pipe ๐ง and resets the timer.
spawnPipe():
- Spawns a pipe ๐ง at a random Y position within a defined range (between lowestPoint and highestPoint).
- The pipe ๐ฎ will always spawn at the same X position but at different Y heights to create variety.
- This script ensures endless pipes ๐ง are spawned at regular intervals to keep the game fun and challenging ๐โโ๏ธ๐ฏ.
Come take a look!
You can find this project and many more in my Github repositories!