๐ฎ VR Racetrack Game ๐๏ธ๐จ
Step into the driverโs seat in this thrilling VR racetrack game! ๐๐จ Control your car ๐ and race around a dynamic track filled with twists and turns. But beware! If your car tips over the track, it will land in the water ๐. Watch as it sinks to the bottom ๐โฌ๏ธ, and donโt worryโyouโll respawn and be back on the track in just 3 seconds โฑ๏ธ๐.
Can you stay on track and race your way to victory? ๐๐
Drive Main Script
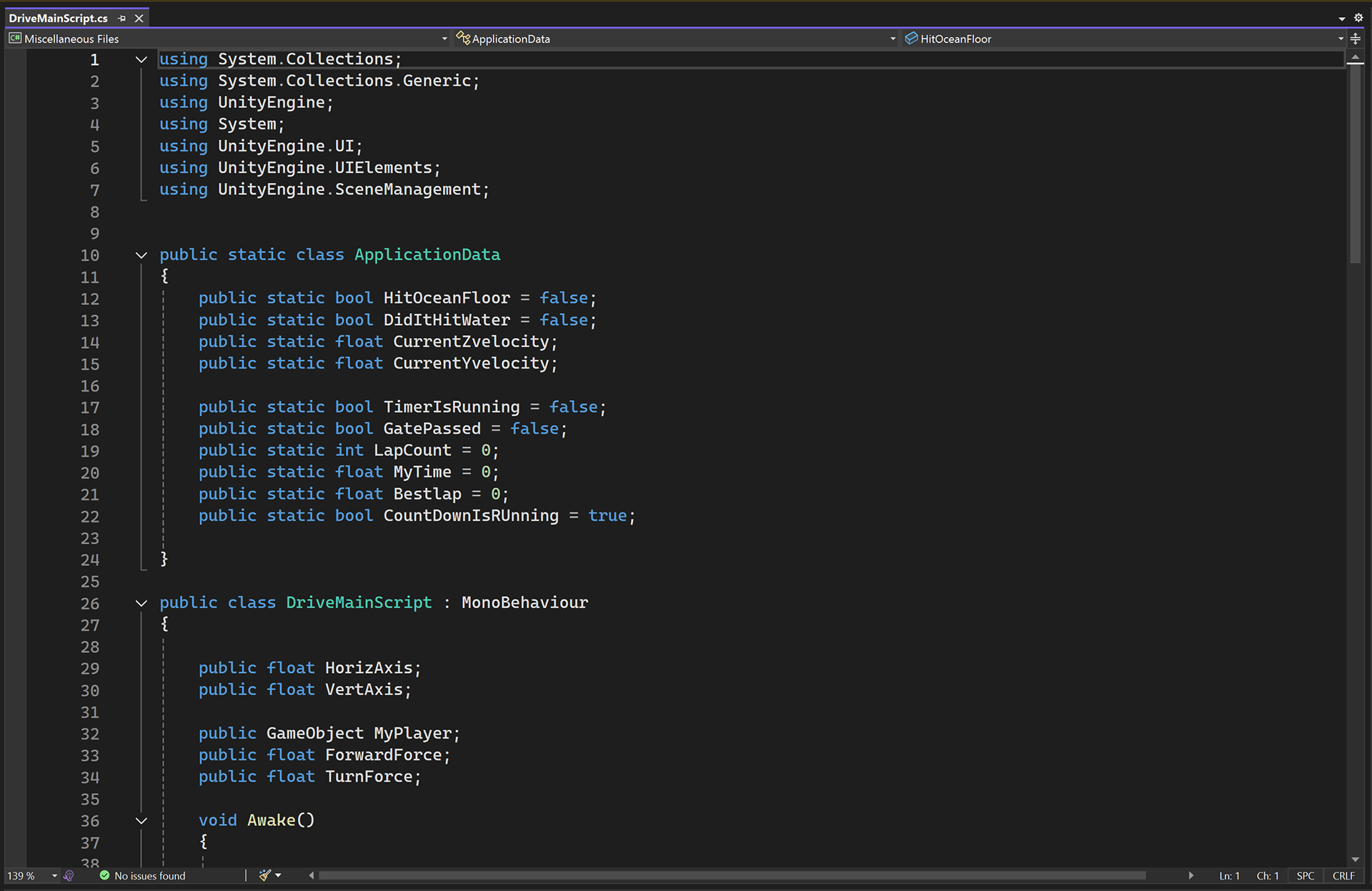
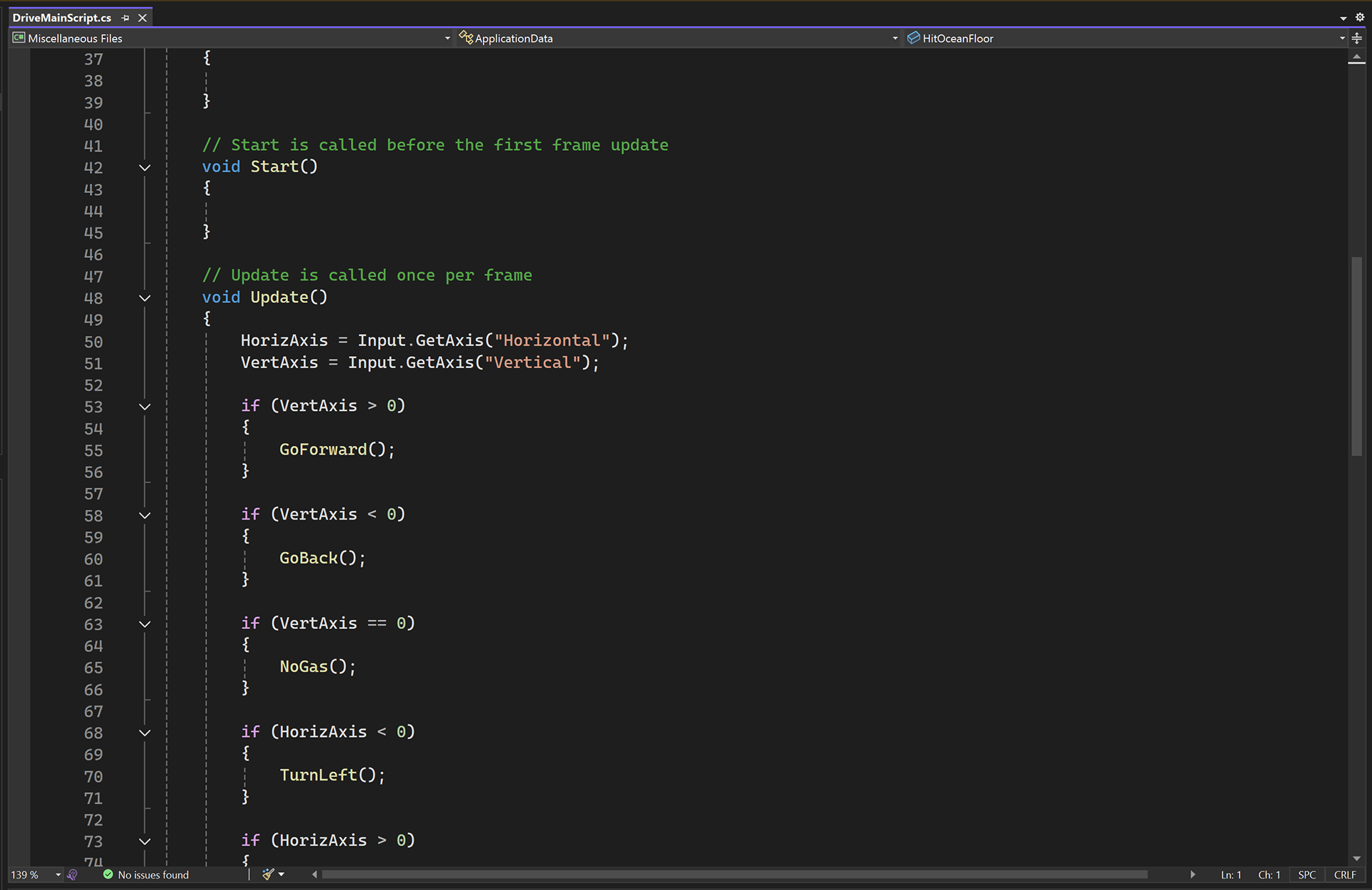
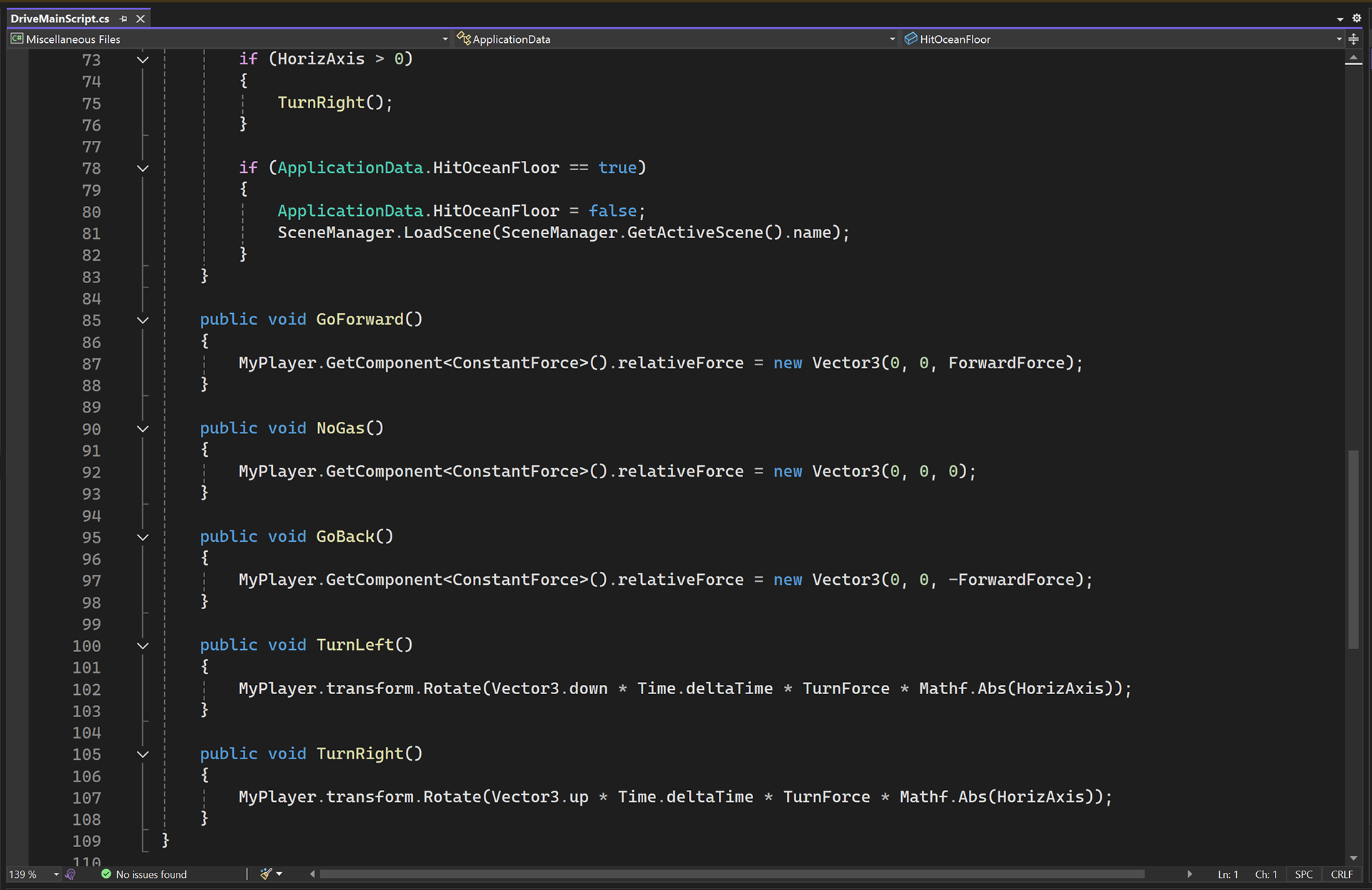
What the Script Does:
This script controls the movement of the player's car ๐๏ธ in a VR racetrack game. It manages the car's acceleration, braking, and turning based on player input. If the car falls into the water ๐ and hits the ocean floor ๐โฌ๏ธ, the scene reloads, and the car respawns.
ApplicationData (Static Class) ๐
This class stores important game data:
- HitOceanFloor: Tracks if the car has hit the ocean floor (i.e., if it falls off the track). ๐โฌ๏ธ
- DidItHitWater: Indicates if the car landed in the water. ๐ฆ
- CurrentZvelocity / CurrentYvelocity: Tracks the car's speed in the Z and Y directions. ๐๏ธ๐จ
- TimerIsRunning: A flag for a timer, likely for race timing. โฑ๏ธ
- GatePassed: Tracks if the player has passed a gate on the track. ๐
- LapCount: Counts the laps completed in the race. ๐
- MyTime: Tracks the current race time. โฒ๏ธ
- Bestlap: Stores the player's best lap time. ๐
- CountDownIsRunning: Indicates if a countdown is active before the race begins. 3... 2... 1... ๐ฆ
- DriveMainScript (Car Controls) ๐๐จ:
Variables:
- HorizAxis / VertAxis: These store the player's input for steering (horizontal) and accelerating/braking (vertical) controls.
- MyPlayer: A reference to the player's car (or vehicle object).
- ForwardForce: The force applied to the car to move it forward. ๐๐จ
- TurnForce: The force applied to rotate (turn) the car. ๐
Key Functions:
- Awake() & Start(): These methods run once when the game starts but are currently empty.
- Update(): This method checks player input every frame. Based on the input:
- Move Forward (Up Arrow/W key): Calls GoForward() ๐๏ธ๐จ
- Move Backward (Down Arrow/S key): Calls GoBack() โฌ๏ธ
- Stop (No Input): Calls NoGas() (stops the car) โ๐๏ธ
- Turn Left (Left Arrow/A key): Calls TurnLeft() ๐โช๏ธ
- Turn Right (Right Arrow/D key): Calls TurnRight() ๐โฉ๏ธ
- If the car hits the ocean floor (falls off the track) ๐โฌ๏ธ, it respawns by reloading the scene. ๐
- GoForward(): Makes the car move forward by applying a force in the Z direction. ๐๏ธ๐จ
- NoGas(): Stops the car by applying zero force in the Z direction. โ
- GoBack(): Makes the car move backward by applying a force in the negative Z direction. โฌ๏ธ๐๏ธ
- TurnLeft(): Rotates the car left by applying a force that turns it to the left. ๐โช๏ธ
- TurnRight(): Rotates the car right by applying a force that turns it to the right. ๐โฉ๏ธ
Start trigger script
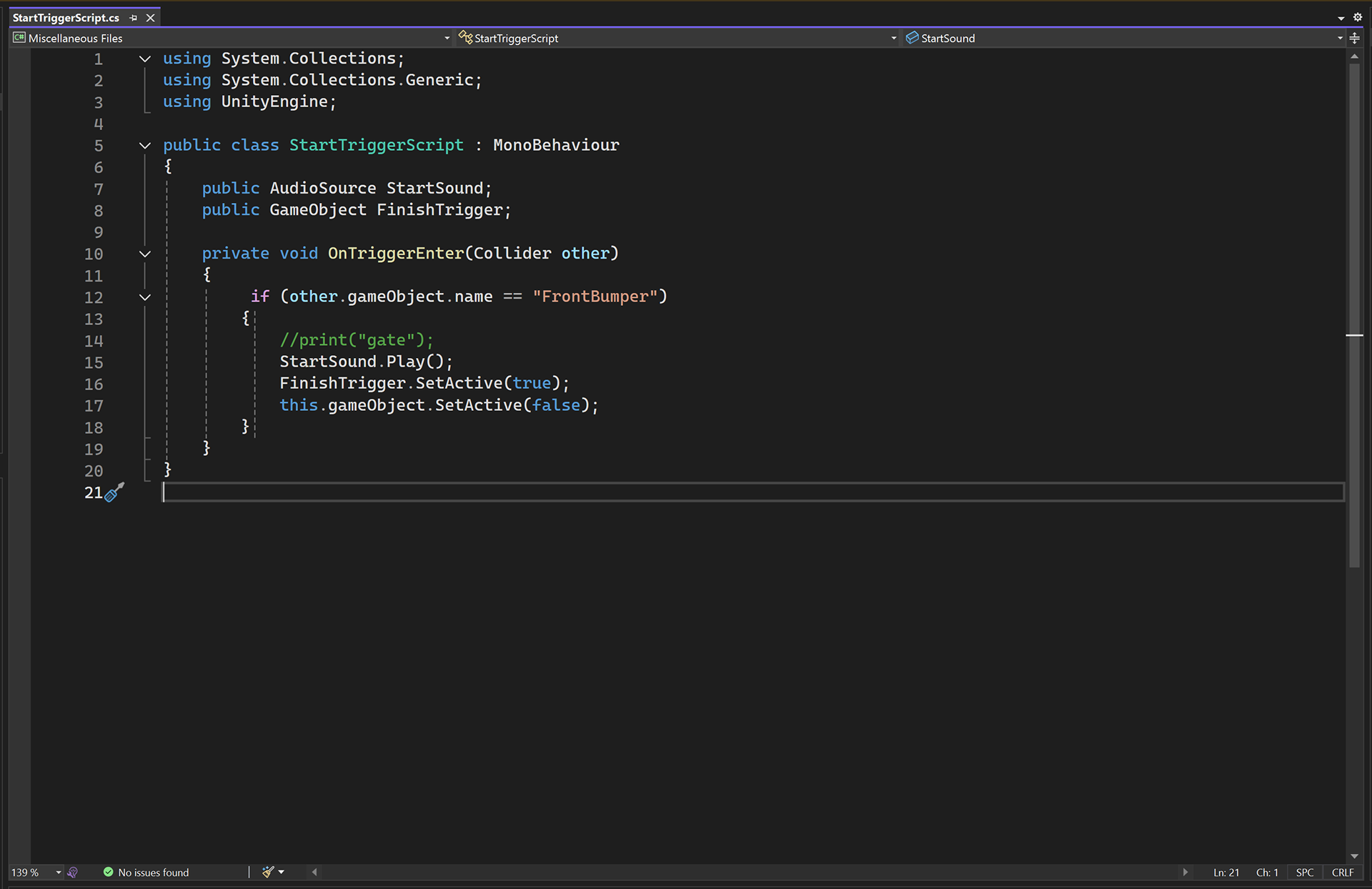
Breakdown ๐ฎ
This script manages the start of a lap in the VR racing game. When the car crosses the start line, it plays a sound and activates the finish line trigger, allowing the player to complete the lap. The start trigger is deactivated after being used.
Key Components
Public Variables:
- StartSound: An AudioSource reference used to play a sound when the player crosses the start line (e.g., a race starting sound or countdown). ๐
- FinishTrigger: A reference to the FinishTrigger game object that will be activated once the start trigger is triggered. ๐ฆ
OnTriggerEnter(Collider other):
- This method is called when another collider enters the trigger zone (the start line).
- It checks if the FrontBumper of the car enters the trigger zone. If true, the player has crossed the start line. ๐๐
Start Line Actions:
- The StartSound is played when the car crosses the start line, indicating the start of a lap. ๐ถ
- The FinishTrigger game object is activated, enabling the player to complete the lap by triggering the finish line when they cross it. ๐ฆ
- The current StartTrigger is deactivated to prevent it from being triggered multiple times. โ
In Summary:
When the player's car crosses the start line (detected by the "FrontBumper"), the script plays a start sound ๐, activates the FinishTrigger for lap completion ๐ฆ, and deactivates itself to ensure it doesn't trigger again. It's part of the race flow, ensuring the player can complete laps one at a time. ๐
Finish Trigger Script
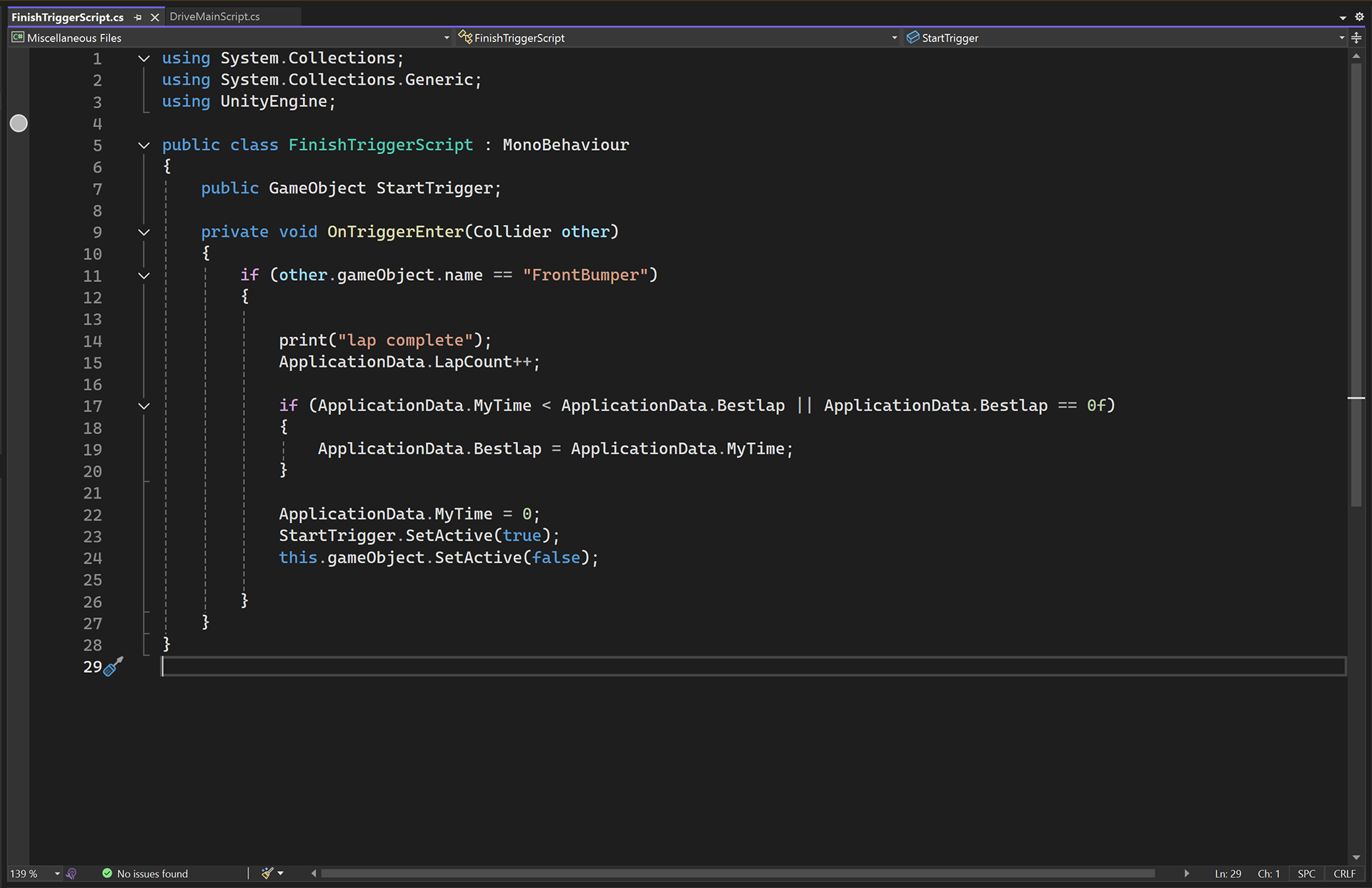
Breakdown ๐
This script handles the logic for completing a lap in a VR racing game. When the car crosses the finish line (detected by a trigger), it increments the lap count ๐, checks if the player's current lap time is a personal best ๐
, and resets the timer for the next lap. It also activates or deactivates the appropriate game objects to control race flow.
Key Components
Public Variable:
- StartTrigger: A reference to the game object that acts as the start trigger for the next lap. This object is activated when the lap is completed. ๐ฆ
OnTriggerEnter(Collider other):
- This method is triggered when another collider enters the trigger zone (the finish line).
- It checks if the car's FrontBumper collider enters the trigger. If so, it means the car has crossed the finish line! ๐๐
Lap Completion:
- The script prints a message: "lap complete" ๐ฃ.
- The LapCount is incremented by 1, keeping track of how many laps the player has completed. ๐ข
- It then checks if the player's current lap time (MyTime) is faster than their Bestlap (the best lap time so far):
- If the current lap is better, Bestlap is updated to the new best time. ๐
- If no best lap has been recorded yet, the script initializes Bestlap with the current lap time. โฑ๏ธ
- MyTime (current lap time) is reset to 0 for the next lap. ๐
StartTrigger Activation:
- The StartTrigger game object is activated (likely showing the next start line or countdown for the next lap). ๐ฆ
- The current FinishTrigger object (the one used to detect lap completion) is deactivated to prevent it from triggering multiple times. โ
HIt Water Splash Script
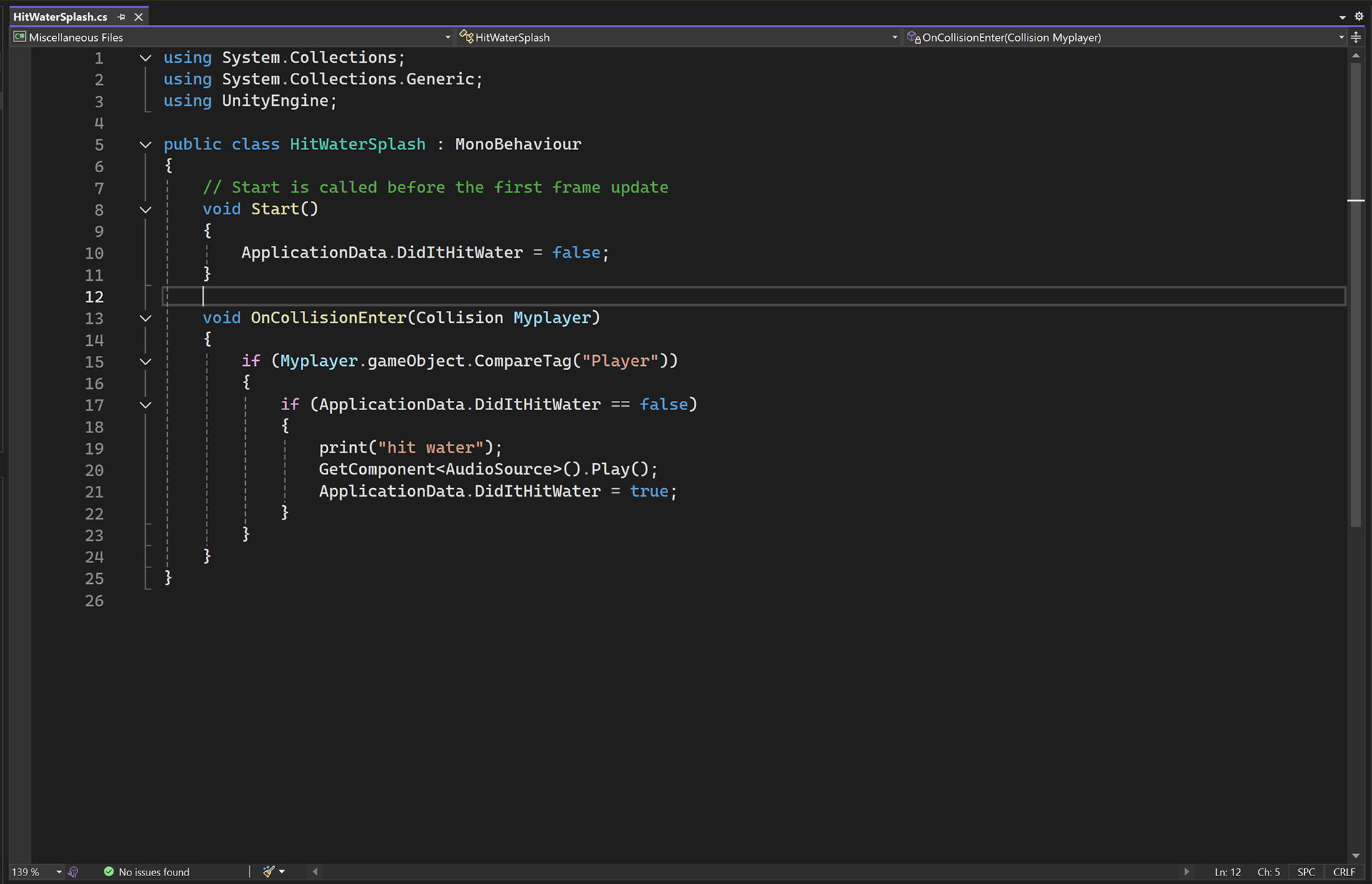
HitWaterSplash Script Breakdown ๐
This script is responsible for triggering a splash sound effect and marking that the player has hit the water when their car collides with the water in your VR racing game.
Key Components
Start Method:
- Initializes the ApplicationData.DidItHitWater to false. This ensures that the game starts with the assumption that the player hasn't hit the water. ๐งโ
OnCollisionEnter(Collision Myplayer):
- This method is called when the car (player) collides with an object that has a Collider attached.
- It checks if the object that collided is tagged as "Player". If true, it proceeds to check if the player has already hit the water. ๐๐ฅ
Water Collision Actions:
- First Collision Check: If the player has not hit the water yet (ApplicationData.DidItHitWater == false), it:
- Prints "hit water" in the console for debugging. ๐ฌ
- Plays the AudioSource component's audio clip (a splash sound effect). ๐ถ๐
- Sets ApplicationData.DidItHitWater to true, indicating that the player has now hit the water. โ
๐ง
Ocean Floor Script
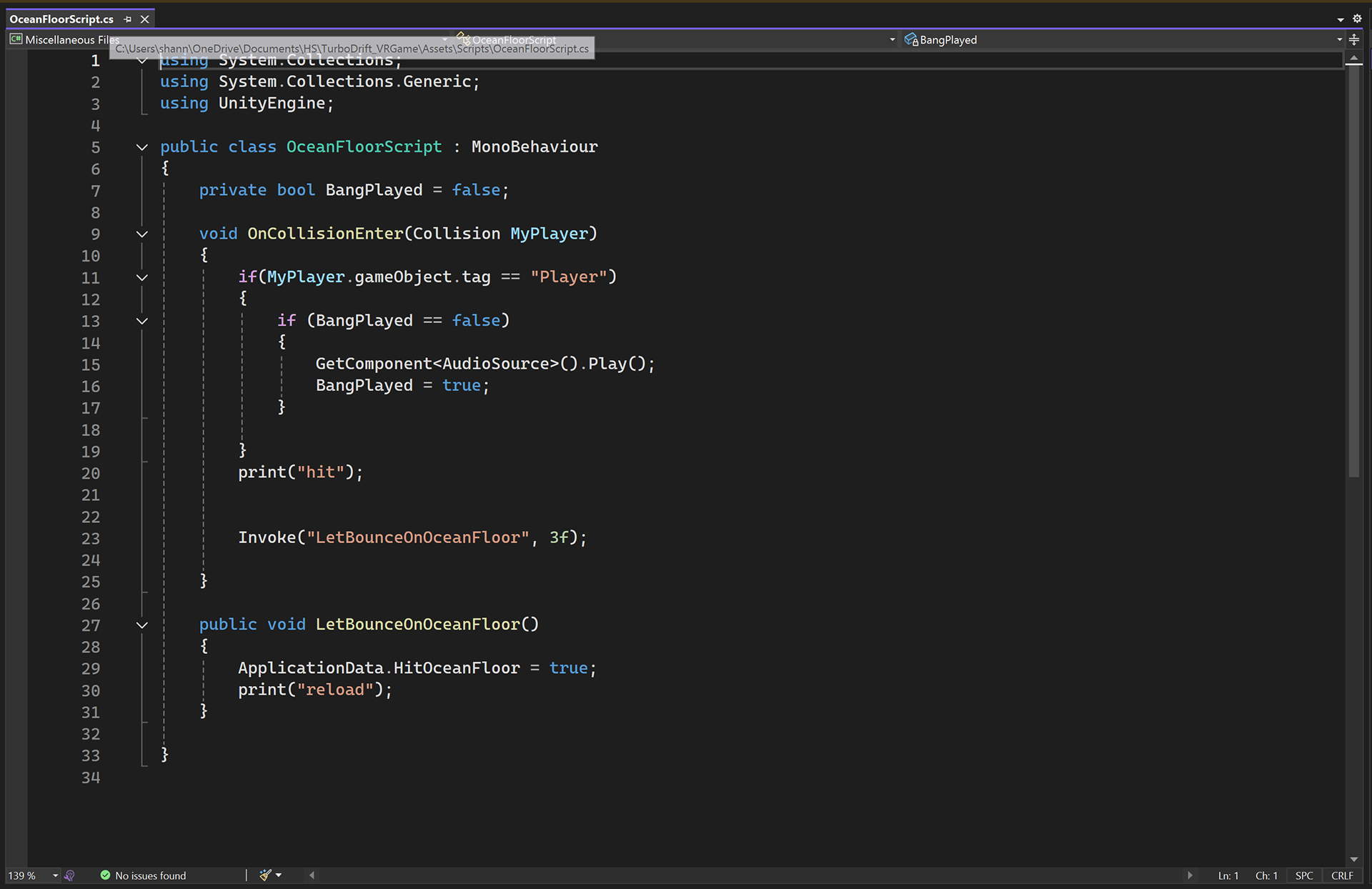
OceanFloorScript Breakdown ๐๐ฅ
This script is responsible for playing a sound effect when the playerโs car collides with the ocean floor and triggering a respawn after 3 seconds if the car hits the bottom of the water.
Key Components:
BangPlayed (bool):
- A flag that tracks whether the sound effect (bang) has already been played upon collision with the ocean floor. ๐๐ฅ
OnCollisionEnter(Collision MyPlayer):
- This method is called when the playerโs car collides with an object that has a Collider.
- It checks if the object involved in the collision is the "Player" by comparing its tag. If the playerโs car hits the ocean floor, it proceeds to play the sound and trigger further actions. ๐๐
Sound Effect (BangPlayed flag):
If the BangPlayed flag is false, it:
- Plays the AudioSource attached to the ocean floor object, which could be a splash or crash sound. ๐ถ๐ฅ
- Sets BangPlayed to true, ensuring the sound effect plays only once until the flag is reset. โ
๐
Invoke("LetBounceOnOceanFloor", 3f):
- This invokes the method LetBounceOnOceanFloor() after 3 seconds, simulating the car bouncing on the ocean floor before respawning. โณ๐๐จ
LetBounceOnOceanFloor():
- This method sets ApplicationData.HitOceanFloor to true, indicating that the playerโs car has hit the ocean floor, and prepares for a respawn.
- It also prints "reload" to the console, signaling that a respawn process is being triggered. ๐๐
Come take a look!
You can find this project and many more in my Github repositories!